This content originally appeared on DEV Community and was authored by Elijah Gathanga
Introduction to Web Development in Go
Web development allows us to create powerful digital solutions across various industries, from e-commerce to social media. While many programming languages can be used for web development, Go offers unique advantages that make it particularly compelling for building robust and high-performance web applications. In this article, we’ll explore why Go is an excellent choice for web development and guide you through setting up a simple web server using Go.
Why choose go for web development
Go offers ideal features which makes it ideal for web development.
1. Performance – Go is a statically typed language and compiled. This means that it is directly converted to machine code thus faster execution time.
2. Concurrency – Go was built with concurrency in mind. With go-routines and channels, you can easily build highly scalable and high performing web applications which can handle many tasks at the same time.
3. Simplicity – It has a clean syntax which is easy to learn and understand.
4. Reduced learning curve – Due to a small set of keywords and concepts in go, the learning curve is significantly reduced.
5. Standard library – Go library has extensive support for building web servers and http requests, thus there is no need for third party libraries.
6. Cross-Platform – Go code once compiled, it can run on other operating systems without being modified.
What you will learn in this article
This guide is perfect for beginners who have just learned Go and want to start building web applications. By the end of this article, you’ll be able to set up a basic web server in Go, handle HTTP requests, and serve HTML templates. note This article assumes you are familiar with go syntax or at least you understand go. If you would like to have a look at go visit to learn more about go syntax. Feel free to check other sites of your choice.
Setting up Go Development Environment
Installing go in your computer
If you have not installed go in your computer, follow the instructions from this and only proceed if Go has successfully been installed.
Choosing an IDE
In this case I will be using a visual studio code editor. In case you want to install it Follow this link and follow instructions. note: Before proceeding to the next ensure you have a text editor and go installed in your machine. It’s very important for the next section.
Setting up the workspace
For Simplicity, we have a relatively straight forward folder structure.
This is the folder structure
There are two main folders: static templates static – will house all our static files like css files, javascript files etc. templates – will house all our html templates. There is also a very important file called main.go. This is the entry point of our program.
Writing your first Go web Server
Now let’s dive into more interesting stuff. The real programming. Before we create our first server, it is important for you to understand how web applications are built. understand concepts like HTTP and how it works. The explanation of how it works is out of scope of this article. If you want to read more about HTTP and its inner workings follow this link. What you should know about HTTP in a nutshell is that it’s a stateless protocol, text-based, request-response protocol that uses the client-server computing model This diagram shows the basic way a web application works.
How Web Application Works
Creating a simple HTTP server
Let’s set up a basic HTTP server in Go. The following code snippet demonstrates how to create a server that listens on port 8080:
Creating a simple server
http.ListenAndServe(":8080", nil)
This code snippet creates a server and listens on port 8080. The method ListenAndServe from the http interface receives a port number to listen to and a handler function. nil – the nil parameter indicates that we are using a default multiplexer.
We have successfully created a server that listens on port 8080.
There are two main parts of a web application;
1. Handler
A handler receives and processes the HTTP request sent from the client. It also calls the template engine to generate the HTML and finally bundles data into the HTTP response to be sent back to the client.
2. Template Engine
A template is code that can be converted into HTML that’s sent back to the client in an HTTP response message. A template can be partly in HTML or not. A template engine generates the final HTML using templates and data. Learn more about Templates
Handling http requests and Routing
Routing is a critical component of any web application, as it determines how requests to various endpoints are handled. In Go, we use the http.HandleFunc function to define these routes.
http.HandleFunc(“/”, Index)
This line of code says that when we request a “/” (this is the root url),handle the path with the function Index. Routing – The net/http package allows you to define routes using http.HandleFunc or http.Handle. The above code snippet creates a route to the home page.
http.HandleFunc creates a rule that invokes the specified function (Index) for requests that match the pattern. This creates the handler We will talk about the Index function later. For now just know that to handle a request, use the above method. Note: This method of handling the requests is if you are using a function to handle a particular pattern
Serving Static files
fsHandler := http.FileServer(http.Dir("./static"))
http.Handle("/static/", http.StripPrefix("/static", fsHandler))
``
We create a handler (**fsHandler**)using the **FileServer** function that serves files from the **static** directory using the **Dir** function. We are going to serve the content from the *static* folder with the URL paths that start with static so that a request for */static/webdev.css*, will be handled as *static/webdev.css*.
At this point we have already set up our **server**, and a **router** which sends requests to their handler functions.
# **HTML templating with Go**
Lets create a simple HTML template to test if our server is working, and execute our template and see if the css is working too.
1. Create a file in *templates* folder and name it **index.html** The content of the file should be as in the figure below. Content of the index.html
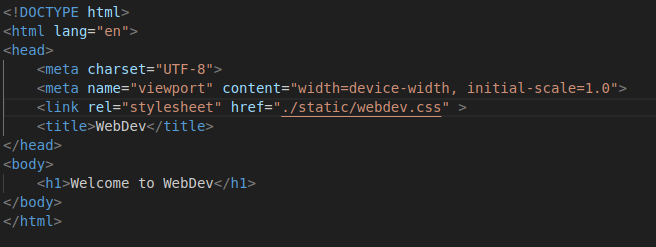
2. Create a function **Index** in the *main.go* file and copy the following contents.
Content of the Index function
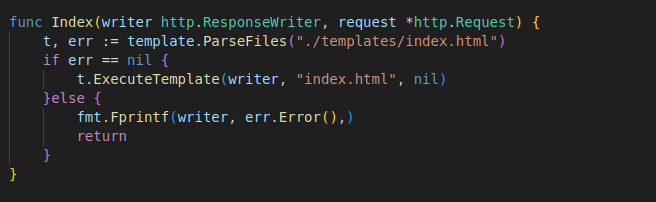
func Index(writer http.ResponseWriter, request *http.Request)
The Index function receives two parameters which are a must. \- **writer** which is of type *response writer*, which writes the response to the client. \- **request** which is a pointer to *http.request* receives a request.
t, err := template.ParseFiles(“./templates/index.html”)
For you to be able to execute a template, you must first load it. You can achieve this using the **ParseFiles** function.
After loading the template file you can now execute it.
t.ExecuteTemplate(writer, “index.html”, nil)
At this point we are ready to have some content in the browser. Now type **localhost:8080** on your favorite browser and you will see something like this.
*Welcome Screen.*
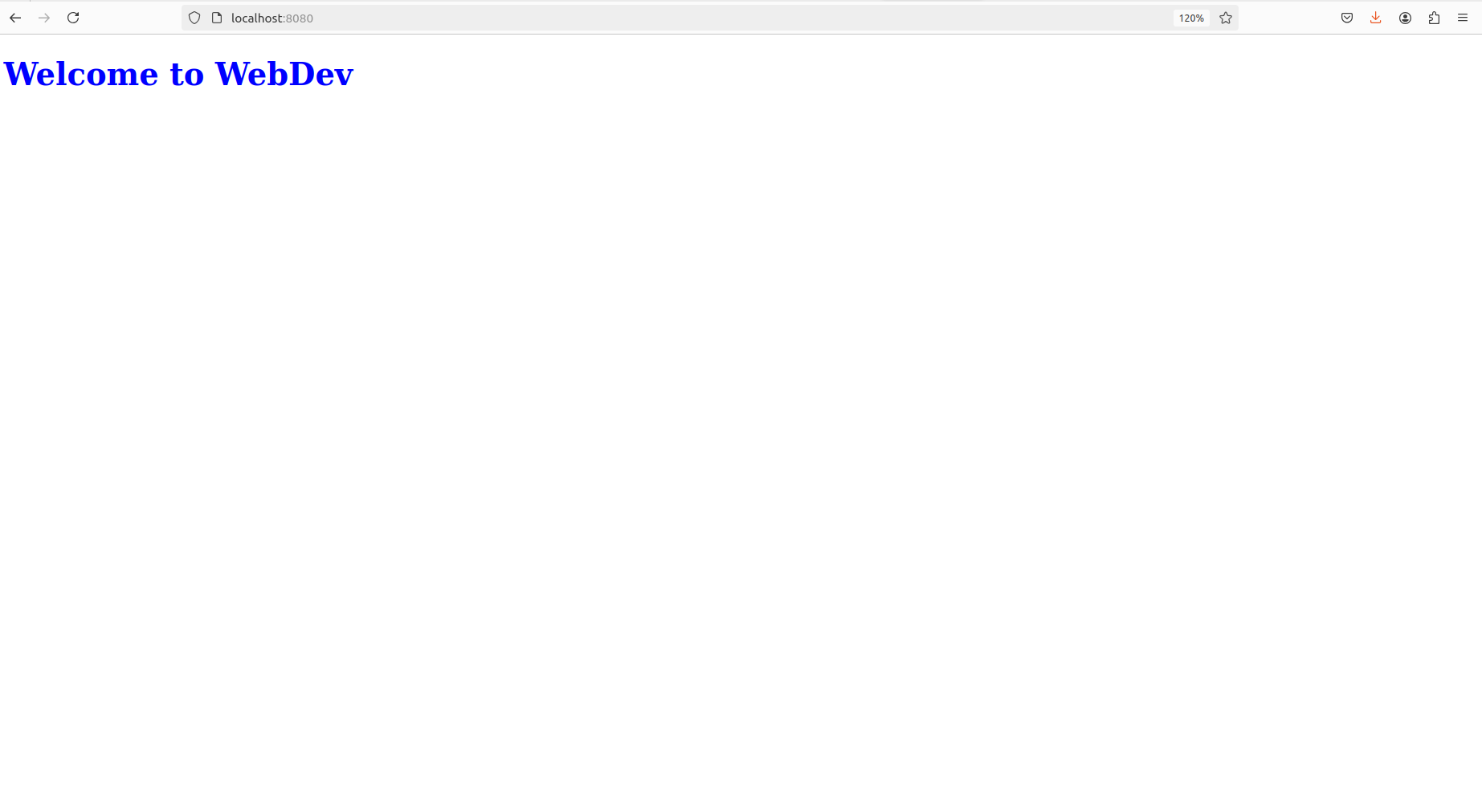
## **More detailed implementation of templating in Go**
Lets create a struct which defines our products and create a slice of product struct. Update your main.go file as follows.
*Updated main.go file*
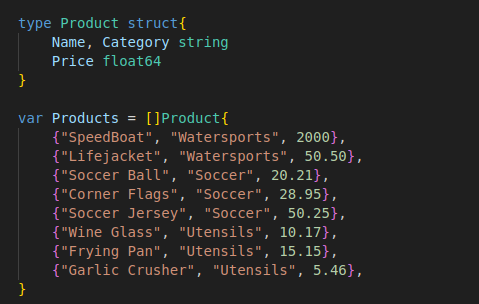
Execute the template now with the products data.
err = indexTemplate.Execute(writer, Products)
if err != nil{
http.Error(writer, “Internal Server Error”, http.StatusInternalServerError)
fmt.Println(“Error Executing Index template”)
return
}
Let’s update the index.html to create a table which will hold the products.
*Updated index.html*
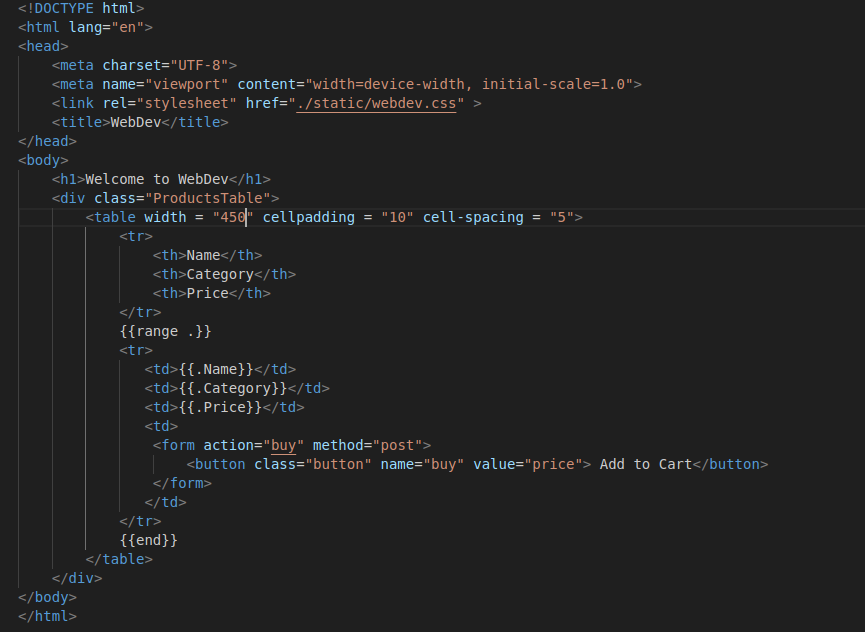
Template Actions are used to generate content from the data that is passed to the **Execute** or **ExecuteTemplate** method. In this case, let’s briefly discuss the template actions used. Template actions are enclosed in double curly braces **{{}}**.
1. {{range .}} \- It iterates through the products slice and adds the content between range and end keywords
2. {{.Name}} \- Inserts the product name in the name column in the table.
3. {{.Category}} \- Inserts the product category in the category column in the table.
4. {{.Price}} \- Inserts the product price in the name price in the table.
5. {{end}} \- marks the end of a block. In this context, templating has helped in **Dynamic Content generation**. We have been able to create a single template for our products slice.
This should be what you see in the browser after the updates.
*Browser with the table*
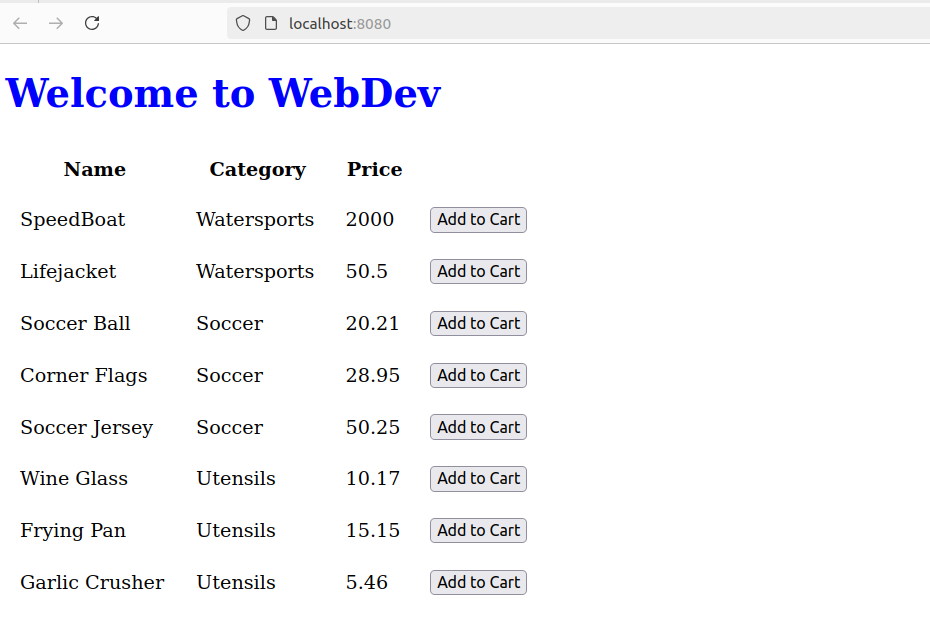
If you don’t have such a screen, ensure you have a similar code as i have provided. If we are on the same page, You can proceed to the next section.
# **Conclusion**
You now know why go will be the next king in web development. Having this brief introduction lets you familiarize with the basic structure you will be using. From this article you have learnt the following;
1\. Creating the go server using **http.ListenAndServe** function. That's how easy it is to create a go server.
2\. Creating routers and handler functions using **http.HandleFunc()**.
3\. Serving the static files using **http.ServeFile()** function.
4\. Parsing and executing template files using **template.Parsefiles()** and **Execute** functions.
This is just getting you started to develop web applications in Golang. ***Coming up…*** \- Creating a Restful API And Database Integration.
***Stay Tuned*** **It's time to get started on the Big idea**
This content originally appeared on DEV Community and was authored by Elijah Gathanga