This content originally appeared on Level Up Coding – Medium and was authored by Naveenkumar Murugan
The story hard core python developer becoming fan of Java
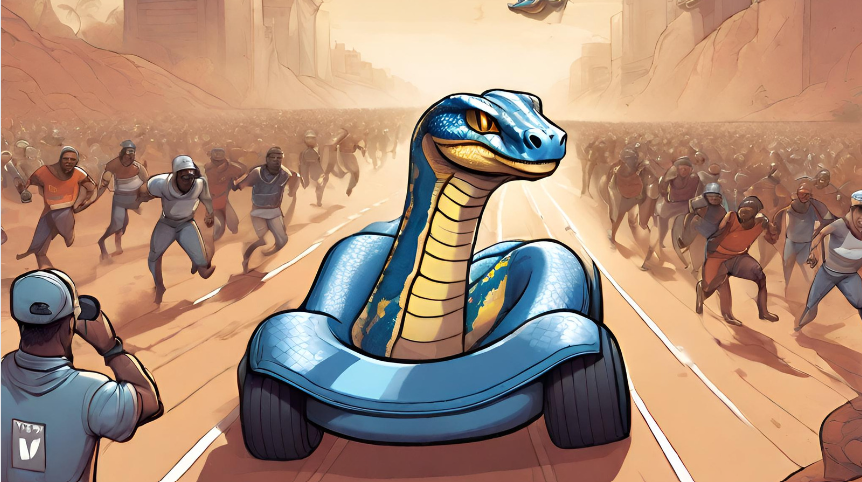
I always been a fan of Python, you can know that from this comment in linkedIn few days ago, whenever there is debate around python over other languages, I blindly suppor tsaying “East or West! python is best” 🙂 but now I am fan of Java too! why? lets see in this article with facts.
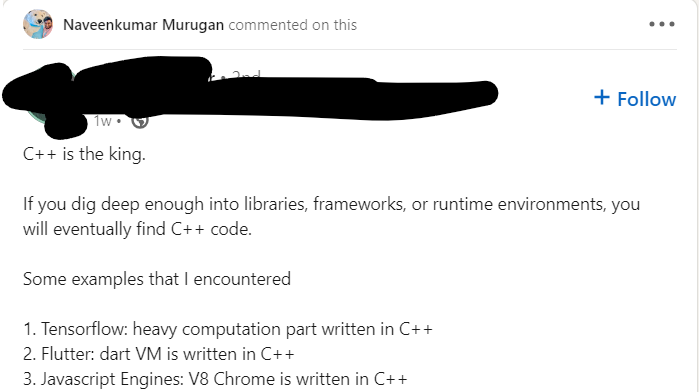
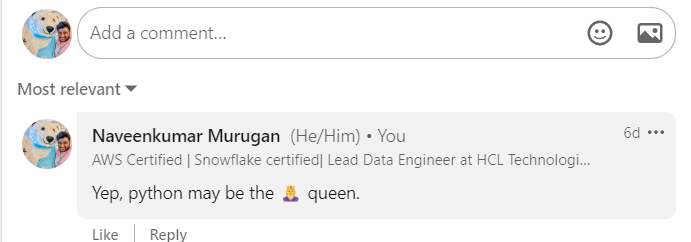
Today (January 14, 2024), I simply wanted to refresh my memory on data structures and algorithms, as it has been a long time. I then aimed to solve the easiest problem first to kick-start my brain waves.
Yes, this is the problem I wanted to solve in Leetcode (https://leetcode.com/problems/running-sum-of-1d-array/description/)
Problem statement (credit: Leetcode)
Given an array nums. We define a running sum of an array as runningSum[i] = sum(nums[0]…nums[i]).
Return the running sum of nums.
Example 1:
Input: nums = [1,2,3,4]
Output: [1,3,6,10]
Explanation: Running sum is obtained as follows: [1, 1+2, 1+2+3, 1+2+3+4].
Common uses of running sums
- Tracking cumulative values: Calculating total sales, expenses, or other values over time.
- Finding patterns and trends: Identifying increasing or decreasing patterns in data.
Although I’ve tried over 10 different solutions (which we’ll explore later in the article) for this problem, I’ve been unable to reduce the runtime below 13 milliseconds using python. I’ve become somewhat frustrated, as the best solution’s runtime is 6 milliseconds (by others) and I’ve been unable to achieve further time reductions.
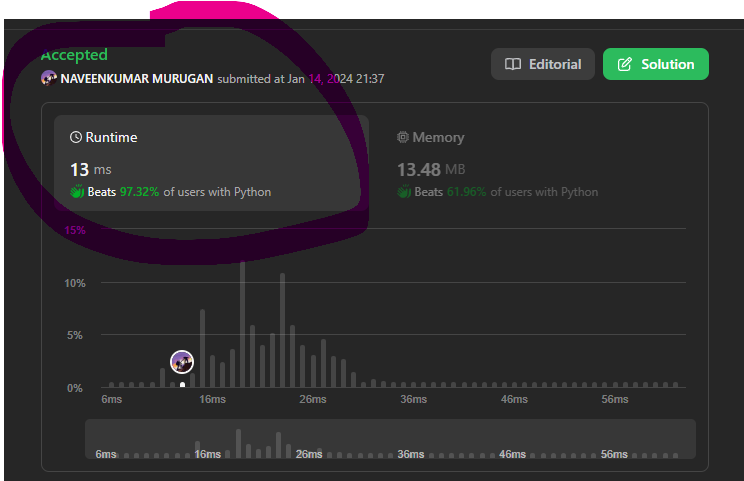
10 different submissions and result:
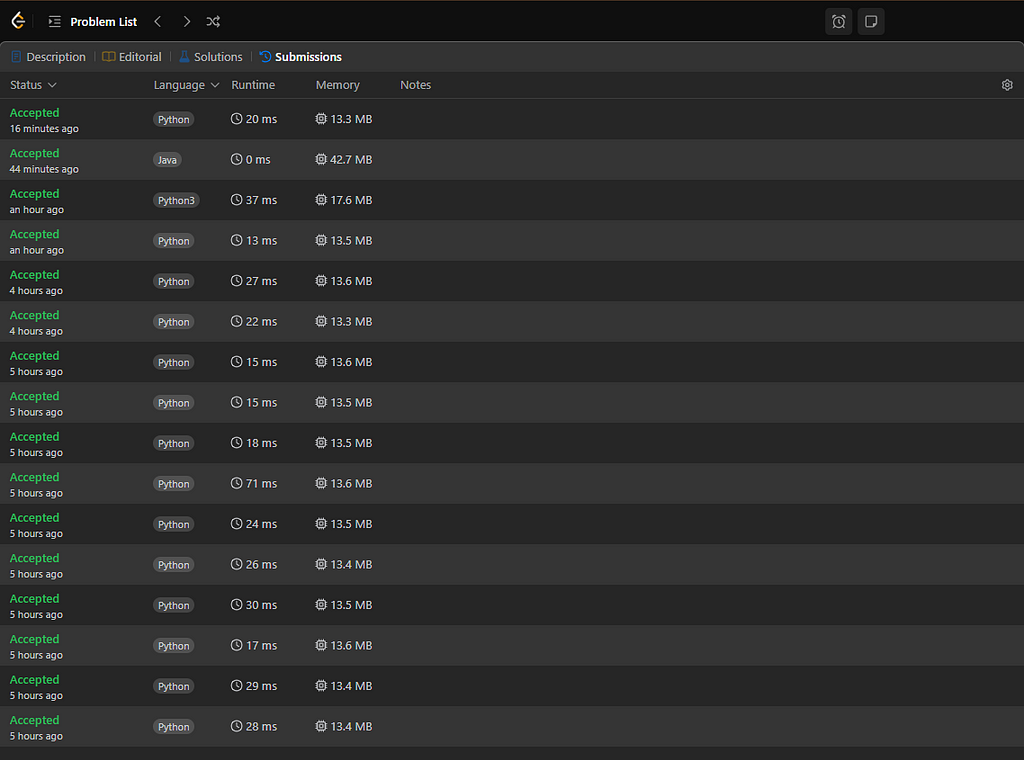
I tried using Python3 (one of the 10 different solution) which supports static typing and make the job easy for the interpreter, but no improvement rather it increased the runtime to 37 milliseconds.
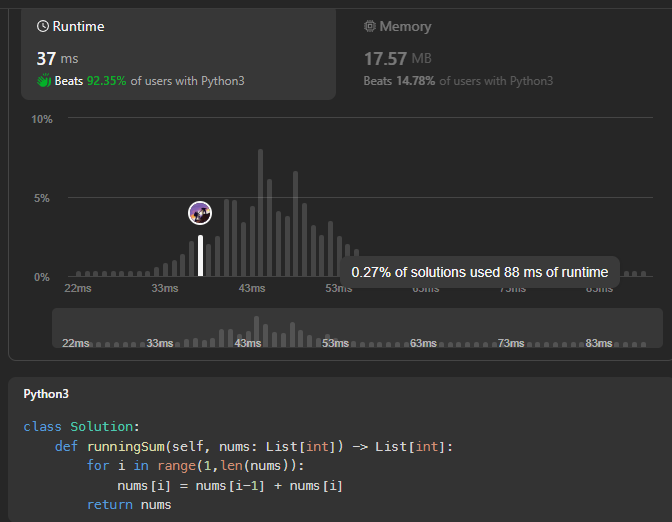
After, trying all the possibilities chosen the best solution based on two points and this time with Java ( same algorithm but Java)
- First element of the runningSUM(the result) going to be the same, as there is element to add on, so started the sum from index 1 rather 0 leaving the first element untouched.
- reused the same list, rather than creating one more new list for storing the result array.
Let us go through the code best solution (You’re Welcome to challenge)
Java solution
class Solution {
public int[] runningSum(int[] nums) {
for (int i = 1; i < nums.length; i++){
nums[i] = nums[i - 1] + nums[i];
}
return nums;
}
}
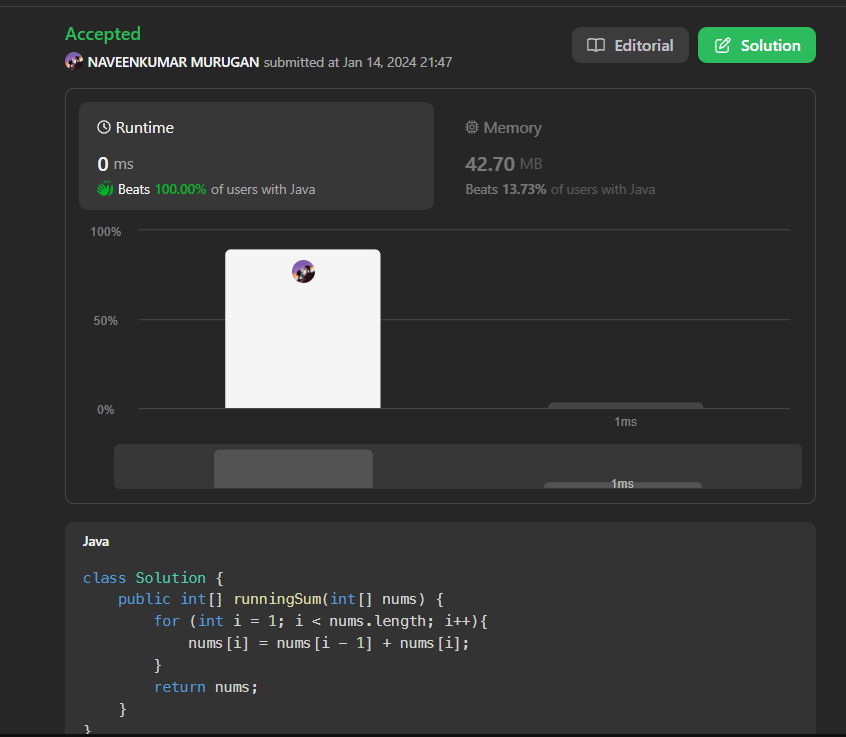
python solution:
class Solution(object):
def runningSum(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
for i in range(1,len(nums)):
nums[i] = nums[i-1] + nums[i]
return nums
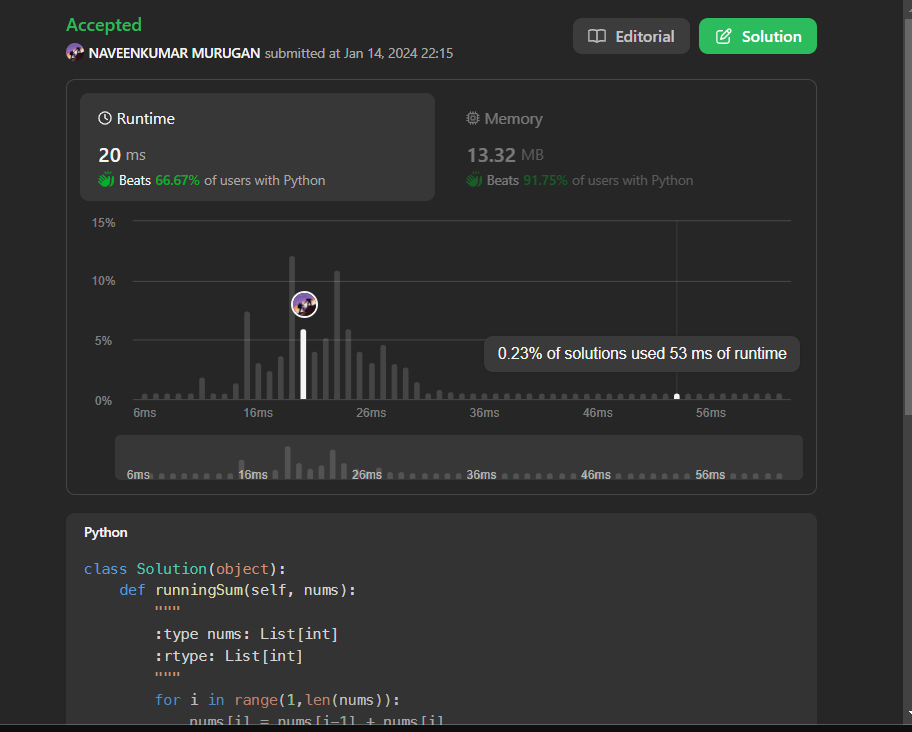
When it comes to speed Java wins, why?
1. Compiled Muscle vs. Interpretive Grace:
- Java: A compiled language, its code is transformed into lean, mean machine code before running. Think optimized instructions for the processor to devour.
- Python: An interpreted language, its code is read line by line like a delicious, but time-consuming, poem. While elegant, this can involve some processing overhead.
2. Typing Tightness Matters:
- Java: Statically typed, it knows all its variable types upfront, allowing for precise optimizations at compile time. No runtime guessing games here!
- Python: Dynamically typed, it figures out variable types on the fly. While flexible, this can lead to additional checks and slow down the party.
3. Memory Management Marvels:
- Python: Uses reference counting, a memory management approach that reclaims memory when objects are no longer referenced. This can be more memory-efficient for certain use cases.
- Java: Employs a more complex garbage collection process with generational algorithms, which can sometimes lead to larger memory usage to manage the heap effectively.
In most of the cases, python was managing memory well 13.32MB but Java 42MB.
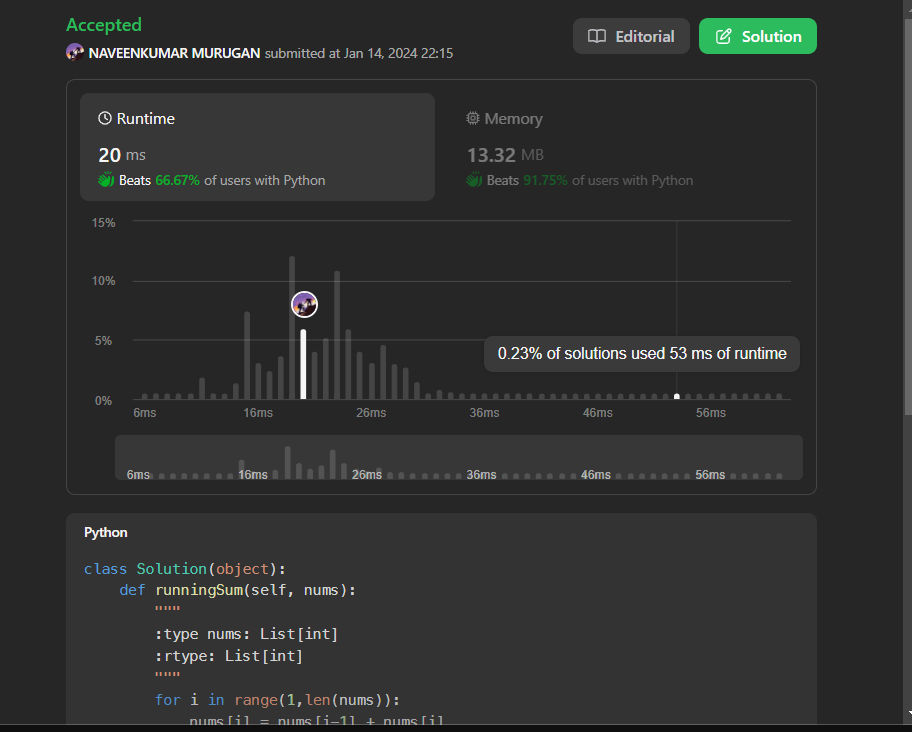
4. Multithreading Magic:
- Java: Champion of multithreading, it effortlessly juggles multiple tasks at once, maximizing processor power. Like a skilled orchestra conductor, it keeps all the threads in perfect harmony.
- Python: The CPython implementation has a Global Interpreter Lock (GIL), which can be a bottleneck for true parallel processing. Think of it as a single microphone for all the instruments, limiting their simultaneous performance.
Remember: While Java often pulls ahead in the speed race, the winner depends on the track (your specific use case). Python’s ease and conciseness might be more valuable in some situations. Ultimately, the best language is the one that gets you to the finish line, whether with blazing speed or graceful agility.
Bonus Tip: Keep an eye on advancements! Both languages are constantly evolving, with innovations like JIT compilation in Python and alternative implementations like Jython and PyPy potentially blurring the performance lines in the future.
By understanding these factors, you can choose the language that best fits your needs, whether you’re chasing lightning-fast applications or seeking elegant solutions. So, buckle up, grab your keyboard, and let the coding adventure begin!
Don’t forget to clap!! Python Squad and Java Squad??????
Extras:
first submission(with enumeration and for loop, sum)
class Solution(object):
def runningSum(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
items = []
for index, num in enumerate(nums):
items.append(sum(nums[0:index+1]))
return items
result:

Second submission(with list comprehension, sum):
class Solution(object):
def runningSum(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
# items = []
# for index, num in enumerate(nums):
# items.append(sum(nums[0:index+1]))
# return items
return [sum(nums[0:index+1]) for index, num in enumerate(nums)]
result:
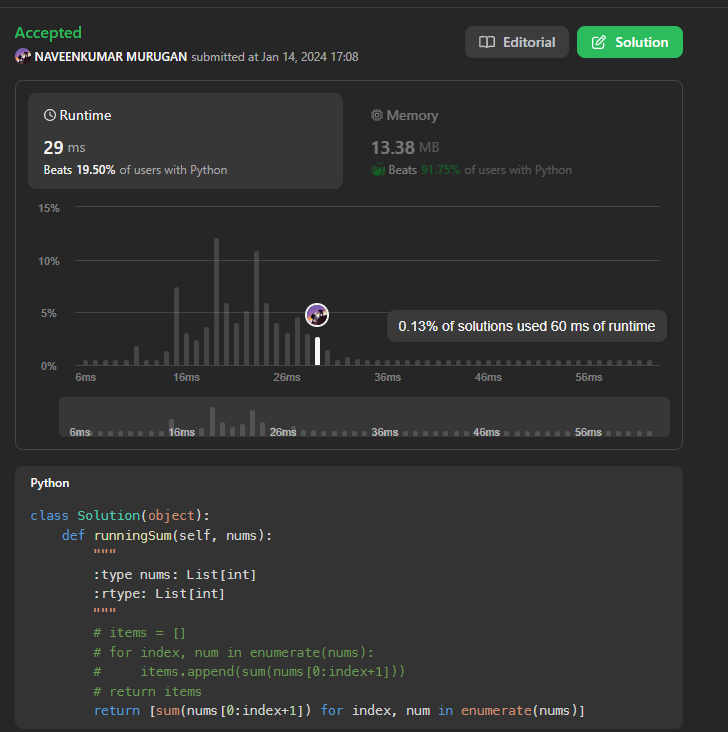
Third(with list comprehension, if else):
class Solution(object):
def runningSum(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
return [num if index == 0 else sum(nums[:index+1]) for index, num in enumerate(nums) ]
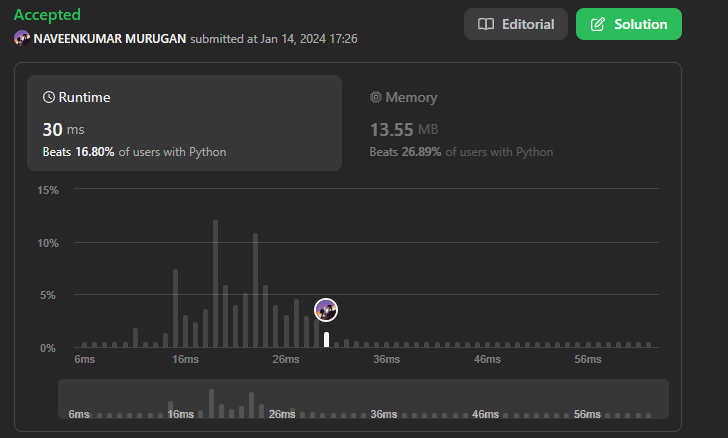
Fourth:
class Solution(object):
def runningSum(self, nums):
"""
:type nums: List[int]
:rtype: List[int]
"""
# items = []
# for index, num in enumerate(nums):
# items.append(sum(nums[0:index+1]))
# return items
# return [sum(nums[0:index+1]) for index, num in enumerate(nums)]
index = 0
items = [nums[0]]
for num in nums[1:]:
items.append(items[-1] + num)
index = index + 1
return items
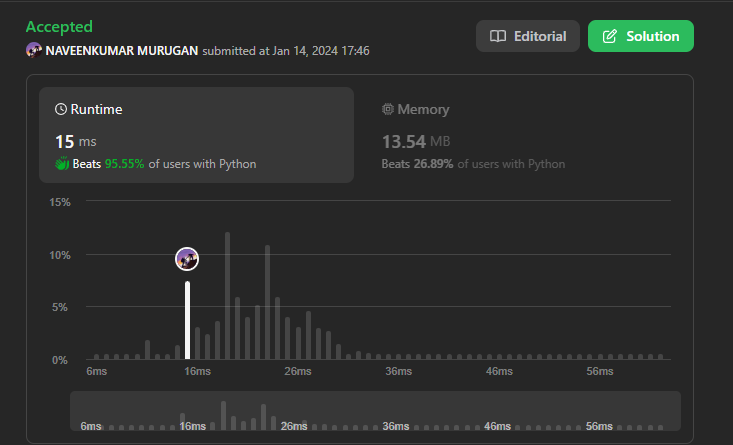
Tired of copy pasting all the soultion, please comment if you need all of them! cheers!
Is Java Truly the Speed King? A Python Developer’s Perspective was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding – Medium and was authored by Naveenkumar Murugan