This content originally appeared on Level Up Coding – Medium and was authored by Chrissie
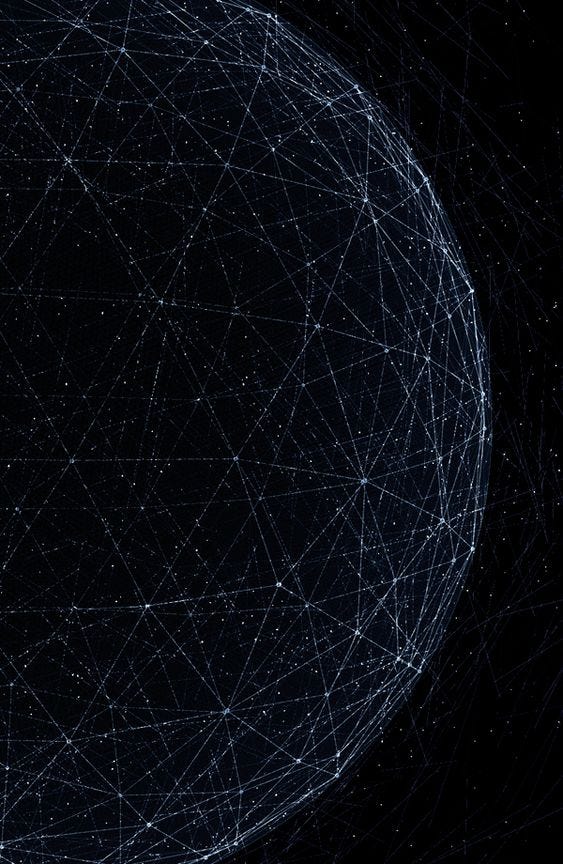
Mastering Pandas: Advanced Techniques for Efficient Data Manipulation
Pandas is a powerful data manipulation library in Python that is widely used by data analysts and scientists to manipulate and analyze large datasets. It provides an easy-to-use interface for data manipulation and is built on top of NumPy, allowing for high-performance computations.
While pandas is well known for its ability to perform basic data manipulation tasks, it also offers a wide range of advanced data manipulation techniques that are not as well-known.
These techniques can help you to wrangle, transform and filter data more efficiently than ever before.
In this article, you will take a deep dive into some of the most advanced data manipulation techniques that pandas has to offer. You will explore everything from grouping and aggregating data to pivot tables, advanced indexing, and slicing techniques. By the end of this episode, you will have a deeper understanding of how to unleash the power of pandas and take your data manipulation skills to the next level!
Introduction to Pandas and its capabilities
Pandas is a powerful and versatile Python library that is widely used for data manipulation and analysis. It provides easy-to-use data structures such as DataFrames and Series, which allow users to efficiently handle and manipulate large datasets. With its rich set of functions and methods, Pandas enables users to clean, transform, and analyze data with ease.
One of the key strengths of Pandas is its ability to handle different types of data, including numerical, text, and categorical data. This makes it a valuable tool for a wide range of data analysis tasks, from simple data cleaning and preprocessing to complex statistical analysis and machine learning.
In addition to its data manipulation capabilities, Pandas also integrates well with other Python libraries such as NumPy, Matplotlib, and Scikit-learn, allowing users to create end-to-end data analysis pipelines seamlessly.
Getting started with Pandas: Installation and Setup
Getting started with Pandas is the first step towards unleashing the power of data manipulation in Python. Before diving into the vast capabilities of this powerful library, it is essential to ensure you have it installed and set up correctly on your system.
To begin, the first step is to install Pandas. This can be easily done using popular package managers like pip or conda. Simply open your command prompt or terminal and run the appropriate command to install Pandas:
Using pip:
“`
pip install pandas
“`
Using conda (if you are using Anaconda distribution):
“`
conda install pandas
“`
Once Pandas is successfully installed, you can start importing it into your Python scripts or Jupyter notebooks. The standard way to import Pandas is by using the conventional alias ‘pd’, which is widely adopted in the data science community:
“`python
import pandas as pd
“`
By importing Pandas with the ‘pd’ alias, you can leverage its functionalities and methods efficiently throughout your data manipulation tasks. With Pandas now set up on your system, you are ready to explore its capabilities and dive into the world of advanced data manipulation techniques.
Loading and reading data into Pandas
Loading and reading data into Pandas is a fundamental step in unleashing the power of this versatile library. Pandas provides various functions to efficiently handle data from different sources like CSV files, Excel spreadsheets, SQL databases, and more.
To load a CSV file into Pandas, you can use the `read_csv()` function, which allows you to quickly read tabular data and store it in a DataFrame, the core data structure in Pandas. This function provides numerous parameters to customize the import process, such as specifying delimiter, header rows, index columns, data types, and more.
Similarly, for Excel files, you can leverage the `read_excel()` function, which simplifies the process of importing data from Excel sheets into Pandas DataFrames. This function supports reading specific sheets, skipping rows, defining column ranges, and handling missing values.
When dealing with SQL databases, Pandas offers the `read_sql()` function, enabling you to execute SQL queries directly against a database and load the results into a DataFrame. This seamless integration with SQL databases allows for easy data manipulation and analysis within the Pandas environment.
By mastering the techniques for loading and reading data into Pandas, you can efficiently work with diverse datasets and unlock the full potential of this powerful data manipulation tool.
1. Loading a CSV File with read_csv():
pythonCopy code
import pandas as pd
# Load CSV file into a Pandas DataFrame
df_csv = pd.read_csv('your_file.csv')
# Display the first few rows of the DataFrame
print(df_csv.head())
2. Loading an Excel File with read_excel():
pythonCopy code
import pandas as pd
# Load Excel file into a Pandas DataFrame
df_excel = pd.read_excel('your_file.xlsx', sheet_name='Sheet1')
# Display the first few rows of the DataFrame
print(df_excel.head())
3. Loading Data from a SQL Database with read_sql():
pythonCopy code
import pandas as pd
import sqlite3
# Create a connection to the SQL database
conn = sqlite3.connect('your_database.db')
# Write your SQL query
query = "SELECT * FROM your_table"
# Load data from SQL query into a Pandas DataFrame
df_sql = pd.read_sql(query, conn)
# Close the database connection
conn.close()
# Display the first few rows of the DataFrame
print(df_sql.head())
Explanation:
- Replace 'your_file.csv', 'your_file.xlsx', 'your_database.db', 'Sheet1', and 'your_table' with the appropriate file name, sheet name, and table name/path for your specific case.
- In the CSV and Excel examples, read_csv() and read_excel() respectively load the data from the specified files into Pandas DataFrames.
- For the SQL example, you first establish a connection to the SQLite database using sqlite3.connect(). Then, you define your SQL query and pass it to pd.read_sql() along with the connection to retrieve data into a DataFrame. Finally, you close the connection.
- Printing df.head() displays the first few rows of the loaded DataFrame to verify that the data has been imported correctly.
Remember to have the necessary libraries installed (pandas, sqlite3 for the SQL example), and replace the file paths, sheet names, and SQL queries with your specific data source information. This should get you started with loading and reading data into Pandas from CSV files, Excel spreadsheets, and SQL databases.
Data cleaning and preprocessing techniques using Pandas
Data cleaning and preprocessing are crucial steps in any data analysis or machine learning project. With the powerful data manipulation capabilities of Pandas, these tasks become much more manageable and efficient.
One common technique in data cleaning is handling missing values. Pandas provides various methods to handle missing data, such as filling missing values with a specific value, interpolating missing values based on existing data, or dropping rows or columns with missing values.
Another important aspect of data preprocessing is handling duplicates in the dataset. Pandas offers functions to identify and remove duplicate rows, ensuring the integrity of the data for analysis.
Furthermore, Pandas allows for data transformation operations such as changing data types, encoding categorical variables, and scaling numerical features. These preprocessing techniques are essential for preparing the data for modeling and analysis.
By mastering data cleaning and preprocessing techniques using Pandas, data scientists and analysts can ensure the quality and reliability of their datasets, leading to more accurate and insightful results in their projects.
1. Handling Missing Values:
pythonCopy code
import pandas as pd
# Load CSV file into a Pandas DataFrame (replace 'your_file.csv' with your file)
df = pd.read_csv('your_file.csv')
# Fill missing values with a specific value (e.g., mean, median, 0)
df_filled = df.fillna(df.mean())
# Interpolate missing values based on existing data
df_interpolated = df.interpolate()
# Drop rows with any missing values
df_dropped_rows = df.dropna()
# Drop columns with any missing values
df_dropped_columns = df.dropna(axis=1)
# Display the first few rows of each DataFrame for comparison
print("Original DataFrame:")
print(df.head())
print("\nDataFrame with Missing Values Filled:")
print(df_filled.head())
print("\nDataFrame with Missing Values Interpolated:")
print(df_interpolated.head())
print("\nDataFrame with Rows Dropped:")
print(df_dropped_rows.head())
print("\nDataFrame with Columns Dropped:")
print(df_dropped_columns.head())
2. Handling Duplicates:
pythonCopy code
import pandas as pd
# Load CSV file into a Pandas DataFrame (replace 'your_file.csv' with your file)
df = pd.read_csv('your_file.csv')
# Identify duplicate rows based on all columns
duplicate_rows = df[df.duplicated()]
# Remove duplicate rows from the DataFrame
df_unique = df.drop_duplicates()
# Display the duplicate rows and the DataFrame with duplicates removed
print("Duplicate Rows:")
print(duplicate_rows)
print("\nDataFrame with Duplicates Removed:")
print(df_unique.head())
3. Data Transformation:
pythonCopy code
import pandas as pd
# Load CSV file into a Pandas DataFrame (replace 'your_file.csv' with your file)
df = pd.read_csv('your_file.csv')
# Change data types of columns (e.g., convert 'date' column to datetime)
df['date'] = pd.to_datetime(df['date'])
# Encode categorical variables using one-hot encoding
df_encoded = pd.get_dummies(df, columns=['category'])
# Scale numerical features (e.g., using Min-Max scaling)
from sklearn.preprocessing import MinMaxScaler
scaler = MinMaxScaler()
df_scaled = pd.DataFrame(scaler.fit_transform(df[['feature1', 'feature2']]), columns=['scaled_feature1', 'scaled_feature2'])
# Display the transformed DataFrame
print("DataFrame with Data Types Changed:")
print(df.dtypes)
print("\nDataFrame with Categorical Variables Encoded:")
print(df_encoded.head())
print("\nDataFrame with Numerical Features Scaled:")
print(df_scaled.head())
Explanation:
- Replace 'your_file.csv' with the path to your dataset file in all examples.
- The first block demonstrates methods for handling missing values: fillna(), interpolate(), and dropna().
- The second block shows how to identify and remove duplicate rows using duplicated() and drop_duplicates().
- The third block includes data transformation techniques:
- Changing data types with pd.to_datetime() for dates.
- Encoding categorical variables using pd.get_dummies().
- Scaling numerical features with MinMaxScaler from scikit-learn.
These examples cover essential data cleaning and preprocessing tasks using Pandas. Adjust the code according to your dataset and preprocessing requirements. This will help you ensure the quality and integrity of your data, making it ready for analysis and machine learning modeling.
Data manipulation and transformation with Pandas
Data manipulation and transformation are at the core of any data analysis or data science project. With Pandas, a powerful Python library, you can unleash a wide range of functionalities to manipulate and transform your data effectively.
One of the key features of Pandas is its ability to handle data in tabular form using DataFrames. This allows for easy manipulation of rows and columns, filtering, sorting, and reshaping data to suit your analysis needs.
With Pandas, you can perform operations such as merging datasets, grouping data, and applying functions to transform your data effortlessly.
Furthermore, Pandas provides powerful tools for data cleaning and preprocessing. You can easily handle missing values, remove duplicates, and convert data types to ensure your data is clean and ready for analysis.
Pandas also offers a wide range of functions for data aggregation, summarization, and transformation, enabling you to derive valuable insights from your data.
Overall, mastering data manipulation and transformation with Pandas can significantly enhance your data analysis workflow and enable you to unlock the full potential of your data.
1. Working with DataFrames:
pythonCopy code
import pandas as pd
# Creating a sample DataFrame
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve'],
'Age': [25, 30, 35, 28, 22],
'Salary': [50000, 60000, 75000, 45000, 70000]
}
df = pd.DataFrame(data)
# Display the original DataFrame
print("Original DataFrame:")
print(df)
# Filtering data based on conditions
df_filtered = df[df['Age'] > 25]
# Sorting the DataFrame by 'Salary' in descending order
df_sorted = df.sort_values(by='Salary', ascending=False)
# Reshaping the DataFrame using pivot
df_pivot = df.pivot(index='Name', columns='Age', values='Salary')
# Display the manipulated DataFrames
print("\nDataFrame Filtered by Age > 25:")
print(df_filtered)
print("\nDataFrame Sorted by Salary (Descending Order):")
print(df_sorted)
print("\nPivoted DataFrame:")
print(df_pivot)
2. Merging and Grouping Data:
pythonCopy code
import pandas as pd
# Creating sample DataFrames for merging
data1 = {
'Name': ['Alice', 'Bob', 'Charlie'],
'Age': [25, 30, 35]
}
data2 = {
'Name': ['David', 'Eve', 'Alice'],
'Salary': [45000, 70000, 50000]
}
df1 = pd.DataFrame(data1)
df2 = pd.DataFrame(data2)
# Merging two DataFrames on 'Name'
df_merged = pd.merge(df1, df2, on='Name')
# Grouping data by 'Age' and calculating average salary
df_grouped = df_merged.groupby('Age')['Salary'].mean().reset_index()
# Display the merged and grouped DataFrames
print("Merged DataFrame:")
print(df_merged)
print("\nGrouped DataFrame with Average Salary by Age:")
print(df_grouped)
3. Data Cleaning and Preprocessing:
pythonCopy code
import pandas as pd
# Creating a sample DataFrame with missing values and duplicates
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David', 'Eve', 'Alice'],
'Age': [25, 30, None, 28, 22, 25],
'Salary': [50000, None, 75000, 45000, 70000, 50000]
}
df = pd.DataFrame(data)
# Handling missing values by filling with mean
df_filled = df.fillna(df.mean())
# Removing duplicate rows
df_unique = df.drop_duplicates()
# Converting 'Age' column to integer type
df['Age'] = df['Age'].astype(int)
# Display the cleaned and transformed DataFrame
print("DataFrame with Missing Values Filled:")
print(df_filled)
print("\nDataFrame with Duplicates Removed:")
print(df_unique)
print("\nDataFrame with 'Age' Column Converted to Integer:")
print(df)
Explanation:
The first block demonstrates basic operations with DataFrames:
- Filtering rows based on conditions with df[df['column'] > value]
- Sorting the DataFrame with df.sort_values(by='column')
- Reshaping the DataFrame using df.pivot(index='index_column', columns='column', values='value_column')
The second block shows how to merge and group data:
- Merging two DataFrames using pd.merge()
- Grouping data with df.groupby('column').function()
The third block covers data cleaning and preprocessing:
- Filling missing values with df.fillna()
- Removing duplicate rows with df.drop_duplicates()
- Converting data types using df['column'].astype()
These examples illustrate how Pandas can be used to manipulate and transform data effectively, including filtering, sorting, merging, grouping, cleaning missing values, removing duplicates, and converting data types. Adjust the code according to your dataset and analysis requirements to harness the full power of Pandas for your data science projects.
Efficient Data Structures in Pandas for Faster Operations
When working with large datasets in Pandas, choosing the right data structures can significantly impact the performance of your operations. By leveraging efficient data structures, you can optimize memory usage and speed up your data manipulation tasks.
One key data structure to consider is the DataFrame. DataFrames are powerful containers for structured data, but they can become memory-intensive when dealing with massive datasets. To enhance performance, consider using efficient data types such as categorical variables or specifying data types explicitly to reduce memory usage.
Another essential data structure in Pandas is the Index. Creating a well-optimized index can speed up operations like data selection, merging, and joining. Utilize multi-level indexes or set appropriate indexing columns to streamline your data access and processing.
Furthermore, leveraging specialized data structures like SparseDataFrame for sparse data or Panel for three-dimensional data can further enhance performance and efficiency in handling complex datasets.
By understanding and utilizing efficient data structures in Pandas, you can boost the speed and scalability of your data processing tasks, ultimately mastering the art of optimizing performance in data analysis and manipulation.
Review of basic data manipulation techniques in Pandas
In this section, we will delve into a review of basic data manipulation techniques in Pandas. Pandas is a powerful Python library that provides data structures and functions to efficiently manipulate and analyze data.
One of the fundamental concepts in Pandas is the DataFrame, which is a two-dimensional labeled data structure with columns of potentially different types. With Pandas, you can easily load data from various sources such as CSV files, Excel spreadsheets, SQL databases, and more into DataFrames for analysis.
Basic data manipulation techniques in Pandas include selecting and filtering data, creating new columns based on existing data, handling missing values, and merging multiple DataFrames. By using methods like `loc`, `iloc`, and boolean indexing, you can extract subsets of data based on specific conditions.
Furthermore, Pandas provides powerful functions for reshaping data, grouping data, and aggregating values. Techniques such as `groupby` and `pivot_table` allow you to perform complex data manipulations and calculations efficiently.
Understanding and mastering these basic data manipulation techniques in Pandas is essential for performing more advanced data analysis and visualization tasks. In the following sections, we will explore advanced techniques that will help you unleash the full power of Pandas for your data manipulation needs.
Understanding hierarchical indexing and multi-level data manipulation
Hierarchical indexing, also known as multi-level indexing, is a powerful feature in pandas that allows for more advanced data manipulation techniques. This feature enables you to work with data that has multiple dimensions or levels, providing a structured way to represent and analyze complex datasets.
By using hierarchical indexing, you can organize and access data in a hierarchical manner, with multiple index levels for rows and columns. This allows for more flexible data manipulation and analysis, especially when dealing with datasets that have multiple dimensions or categories.
One key benefit of hierarchical indexing is the ability to perform operations on subsets of data at different levels of the index. This means you can easily aggregate or slice data based on specific levels of the index, making it easier to extract insights and perform complex calculations.
Overall, understanding hierarchical indexing and mastering multi-level data manipulation techniques in pandas can greatly enhance your data analysis capabilities and help you unlock the full potential of your datasets.
1. Creating a DataFrame with Hierarchical Indexing:
pythonCopy code
import pandas as pd
# Creating a sample DataFrame with hierarchical index
data = {
'Value': [10, 20, 30, 40, 50, 60],
'Category': ['A', 'B', 'A', 'B', 'A', 'B'],
'Subcategory': ['X', 'Y', 'X', 'Y', 'X', 'Y']
}
index = pd.MultiIndex.from_tuples([('Group1', 1), ('Group1', 2), ('Group2', 1), ('Group2', 2), ('Group3', 1), ('Group3', 2)],
names=['Group', 'ID'])
df = pd.DataFrame(data, index=index)
# Display the DataFrame with hierarchical index
print("DataFrame with Hierarchical Index:")
print(df)
2. Indexing and Slicing with Hierarchical Index:
pythonCopy code
# Selecting data using loc with hierarchical index
subset1 = df.loc['Group1']
# Selecting data from specific levels of the index
subset2 = df.loc[('Group1', 1)]
# Slicing data from multiple levels of the index
subset3 = df.loc[('Group1', 1):('Group2', 2)]
# Display the subsets of the DataFrame
print("\nSubset of Group1:")
print(subset1)
print("\nSubset of (Group1, 1):")
print(subset2)
print("\nSubset from (Group1, 1) to (Group2, 2):")
print(subset3)
3. Aggregation and Calculations with Hierarchical Index:
pythonCopy code
# Aggregating data at different levels of the index
mean_by_group = df.groupby('Group').mean()
# Performing calculations on subsets of data
total_by_category = df.groupby('Category')['Value'].sum()
# Display the aggregated results
print("\nMean Value by Group:")
print(mean_by_group)
print("\nTotal Value by Category:")
print(total_by_category)
Explanation:
- The first block creates a sample DataFrame with hierarchical indexing using pd.MultiIndex.from_tuples().
- The second block demonstrates indexing and slicing techniques:
- Using df.loc['Group1'] to select data for a specific level of the index.
- Using df.loc[('Group1', 1)] to select data for a specific combination of index values.
- Slicing with df.loc[('Group1', 1):('Group2', 2)] to extract a range of data.
- The third block showcases aggregation and calculations:
- Using df.groupby('Group').mean() to calculate the mean value for each group.
- Using df.groupby('Category')['Value'].sum() to calculate the total value for each category.
These examples illustrate how to create, manipulate, slice, and aggregate data using hierarchical indexing in Pandas. Hierarchical indexing allows for more structured and flexible data analysis, especially for datasets with multiple dimensions or categories. Adjust the code according to your dataset and analysis requirements to leverage the power of multi-level data manipulation techniques in Pandas.
Exploring advanced data reshaping methods
In the world of data manipulation, mastering advanced techniques for reshaping data can significantly enhance your analytical capabilities. One such powerful tool at your disposal is the Pandas library in Python. By leveraging Pandas, you can efficiently reshape your data to suit your analysis needs, leading to clearer insights and more effective decision-making.
One advanced data reshaping method worth exploring is the pivot table. This technique allows you to reorganize your data, summarizing and aggregating information according to specified criteria. By pivoting your data, you can transform rows into columns, group data by different variables, and perform calculations to extract valuable insights.
Another valuable technique is the melt function, which enables you to reshape your data from wide to long format or vice versa. This flexibility is especially useful when dealing with datasets that require restructuring to facilitate analysis or visualization.
Furthermore, understanding multi-indexing in Pandas can unlock even more powerful data reshaping capabilities. By creating hierarchical indexes, you can organize and manipulate multi-dimensional data with ease, enabling you to perform complex analyses and generate comprehensive reports.
Incorporating these advanced data reshaping methods into your data manipulation toolkit can elevate your analytical skills and empower you to extract meaningful insights from your datasets. By mastering these techniques, you can unleash the full potential of Pandas and take your data analysis to the next level.
Leveraging groupby operations for insightful data analysis
Groupby operations in pandas are a powerful tool for unlocking valuable insights from your data. By grouping your data based on specific criteria and then applying functions to these groups, you can quickly analyze trends, patterns, and relationships within your dataset.
One of the key benefits of using groupby operations is the ability to aggregate data efficiently. Whether you want to calculate the sum, mean, count, or any other statistical metric for each group, pandas makes it easy to perform these calculations in just a few lines of code.
Moreover, groupby operations enable you to perform complex data manipulations and transformations with ease. You can apply custom functions to each group, filter out specific groups based on certain conditions, or even create new columns based on group-level calculations.
Overall, mastering the art of leveraging groupby operations in pandas can take your data analysis skills to the next level, allowing you to uncover hidden insights and make informed decisions based on your findings.
1. Creating a DataFrame for Groupby Operations:
pythonCopy code
import pandas as pd
# Creating a sample DataFrame
data = {
'Category': ['A', 'B', 'A', 'B', 'A', 'B'],
'Value1': [10, 20, 30, 40, 50, 60],
'Value2': [100, 200, 300, 400, 500, 600]
}
df = pd.DataFrame(data)
# Display the original DataFrame
print("Original DataFrame:")
print(df)
2. Applying Groupby and Aggregation Functions:
pythonCopy code
# Grouping data by 'Category' and calculating mean for each group
mean_by_category = df.groupby('Category').mean()
# Calculating sum of 'Value1' for each group
sum_value1 = df.groupby('Category')['Value1'].sum()
# Counting the number of rows in each group
count_by_category = df.groupby('Category').size()
# Display the aggregated results
print("\nMean Value by Category:")
print(mean_by_category)
print("\nTotal Value1 by Category:")
print(sum_value1)
print("\nCount of Rows by Category:")
print(count_by_category)
3. Applying Custom Functions and Transformations:
pythonCopy code
# Define a custom function to calculate the difference between max and min
def difference_func(x):
return x.max() - x.min()
# Applying the custom function to each group
difference_by_category = df.groupby('Category')['Value2'].agg(difference_func)
# Filtering out groups with sum of 'Value1' greater than a threshold
filtered_groups = df.groupby('Category').filter(lambda x: x['Value1'].sum() > 80)
# Creating a new column with group-level calculations
df['Value1_Sum_By_Category'] = df.groupby('Category')['Value1'].transform('sum')
# Display the results of custom functions and transformations
print("\nDifference between Max and Min Value2 by Category:")
print(difference_by_category)
print("\nDataFrame after Filtering Groups:")
print(filtered_groups)
print("\nDataFrame with New Column 'Value1_Sum_By_Category':")
print(df)
Explanation:
The first block creates a sample DataFrame for groupby operations.
The second block demonstrates basic aggregation functions:
- Using df.groupby('Category').mean() to calculate the mean value for each category.
- Using df.groupby('Category')['Value1'].sum() to calculate the sum of 'Value1' for each category.
- Using df.groupby('Category').size() to count the number of rows in each category.
The third block shows more advanced operations:
- Defining a custom function difference_func() to calculate the difference between max and min values, and applying it with df.groupby('Category')['Value2'].agg(difference_func).
- Filtering out groups based on a condition with df.groupby('Category').filter(lambda x: x['Value1'].sum() > 80).
- Creating a new column ‘Value1_Sum_By_Category’ with group-level sum using df.groupby('Category')['Value1'].transform('sum').
These examples illustrate how to use groupby operations in Pandas to perform aggregation, apply custom functions, filter groups, and create new columns based on group-level calculations. Adjust the code according to your dataset and analysis requirements to gain valuable insights and make informed decisions from your data.
Utilizing merge and join functions for combining datasets
Merge and join functions are powerful tools in a data scientist’s toolbox for combining datasets seamlessly and efficiently. These functions allow you to bring together different datasets based on common columns or indices, enabling you to create a comprehensive and enriched dataset for analysis.
By utilizing merge and join functions in Pandas, you can perform various types of joins such as inner join, outer join, left join, and right join to merge datasets based on specific criteria. This flexibility gives you the freedom to choose the merging strategy that best suits your analysis requirements.
Moreover, merge and join functions in Pandas enable you to handle missing or redundant data gracefully. You can specify how to handle missing values, duplicate entries, and conflicting data during the merging process, ensuring the integrity and accuracy of your final dataset.
In summary, mastering the merge and join functions in Pandas empowers you to combine datasets effectively, unlock valuable insights from diverse sources of data, and elevate your data manipulation skills to a more advanced level.
1. Creating Sample DataFrames for Merge and Join:
pythonCopy code
import pandas as pd
# Creating two sample DataFrames to demonstrate merge and join
data1 = {
'ID': [1, 2, 3, 4],
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Age': [25, 30, 35, 28]
}
data2 = {
'ID': [1, 2, 3, 5],
'Salary': [50000, 60000, 75000, 45000]
}
df1 = pd.DataFrame(data1)
df2 = pd.DataFrame(data2)
# Display the original DataFrames
print("DataFrame 1:")
print(df1)
print("\nDataFrame 2:")
print(df2)
2. Performing Different Types of Joins:
pythonCopy code
# Performing an inner join on 'ID' column
inner_join = pd.merge(df1, df2, on='ID', how='inner')
# Performing a left join on 'ID' column
left_join = pd.merge(df1, df2, on='ID', how='left')
# Performing an outer join on 'ID' column
outer_join = pd.merge(df1, df2, on='ID', how='outer')
# Performing a right join on 'ID' column
right_join = pd.merge(df1, df2, on='ID', how='right')
# Display the results of different types of joins
print("\nInner Join:")
print(inner_join)
print("\nLeft Join:")
print(left_join)
print("\nOuter Join:")
print(outer_join)
print("\nRight Join:")
print(right_join)
3. Handling Missing Values and Conflicting Data:
pythonCopy code
# Creating sample DataFrames with conflicting data
data3 = {
'ID': [1, 2, 3, 4],
'Name': ['Alice', 'Bob', 'Charlie', 'Eve'],
'Age': [25, 30, 35, 22]
}
df3 = pd.DataFrame(data3)
# Performing a merge with conflicting data and handling duplicates
merged_data = pd.merge(df1, df3, on='ID', suffixes=('_df1', '_df3'))
# Display the result with conflicting data and suffixes
print("\nMerged DataFrame with Conflicting Data:")
print(merged_data)
Explanation:
The first block creates two sample DataFrames df1 and df2 for demonstration purposes.
The second block demonstrates different types of joins:
- Inner join using pd.merge(df1, df2, on='ID', how='inner').
- Left join using pd.merge(df1, df2, on='ID', how='left').
- Outer join using pd.merge(df1, df2, on='ID', how='outer').
- Right join using pd.merge(df1, df2, on='ID', how='right').
The third block shows handling conflicting data and specifying suffixes:
- Creating a third DataFrame df3 with conflicting data.
- Merging df1 and df3 with pd.merge() and handling conflicting column names with suffixes=('_df1', '_df3').
These examples illustrate how to use merge and join functions in Pandas to combine datasets based on common columns (ID in this case) and handle different types of joins (inner, outer, left, right). Adjust the code according to your dataset and merging requirements to create comprehensive datasets for analysis while managing missing values, duplicates, and conflicting data effectively.
Implementing vectorized operations and custom functions for efficient data processing
Implementing vectorized operations and custom functions is a powerful technique for efficient data processing when working with pandas. By leveraging vectorized operations, you can apply operations to entire arrays or columns of data at once, rather than iterating through each element individually. This approach not only simplifies your code but also speeds up the execution process, making it a key strategy for optimizing performance in Pandas.
Custom functions allow you to define complex operations tailored to your specific data processing needs. By creating custom functions, you can encapsulate logic that may be reused across different parts of your data analysis pipeline. This not only helps in streamlining your code but also improves readability and maintainability.
When combining vectorized operations with custom functions, you can unlock the full potential of pandas for advanced data manipulation. This approach enables you to efficiently process and transform your data, making it easier to derive valuable insights and make informed decisions based on your analysis. By mastering these techniques, you can take your data manipulation skills to the next level and unleash the power of pandas in your data projects.
Handling missing data and outliers effectively
Handling missing data and outliers effectively is crucial in ensuring the integrity and accuracy of your data analysis. Missing data can significantly impact the results of your analysis if not dealt with properly. There are various techniques to handle missing data, such as imputation methods like mean, median, or mode imputation, or more advanced techniques like predictive modeling to estimate missing values.
Outliers, on the other hand, can skew your analysis and lead to inaccurate results if not addressed appropriately. It is important to identify outliers using statistical methods like Z-scores or visualization techniques such as box plots and scatter plots. Once identified, outliers can be handled by either removing them if they are erroneous data points or transforming them using techniques like winsorization or robust regression.
By effectively handling missing data and outliers in your dataset, you can ensure that your data analysis is robust and reliable, enabling you to draw accurate insights and make informed decisions based on the data.
Optimizing performance with efficient coding practices and parallel processing
Optimizing performance is crucial when working with large datasets in Python using Pandas. By implementing efficient coding practices and utilizing parallel processing, you can significantly enhance the speed and efficiency of your data manipulation tasks.
One key aspect of optimizing performance is to leverage vectorized operations in Pandas. Instead of iterating through rows one by one, which can be slow, vectorized operations apply operations to entire arrays of data at once. This approach takes advantage of Pandas’ underlying NumPy capabilities for faster computation.
Another technique to improve performance is to minimize memory usage. This can be achieved by selecting only the necessary columns or rows for your analysis, avoiding unnecessary data loading and processing. Additionally, using data types that consume less memory, such as integers instead of floats, can help reduce memory usage and speed up calculations.
Parallel processing is another powerful tool for optimizing performance. By splitting your data into chunks and processing them concurrently using multiple CPU cores, you can achieve significant speed improvements for computationally intensive tasks. Libraries like Dask and joblib can help you implement parallel processing in Pandas with ease.
By incorporating efficient coding practices, leveraging vectorized operations, minimizing memory usage, and implementing parallel processing techniques, you can unleash the full power of Pandas for advanced data manipulation and analysis. These strategies will not only improve the performance of your data workflows but also enable you to work with larger datasets more effectively.
Memory Management Tips to Reduce Overhead in Pandas
Memory management is crucial when working with large datasets in Pandas to optimize performance and reduce overhead. One effective strategy is to minimize unnecessary memory usage by selecting the appropriate data types for columns. For example, using int8 instead of int64 for integer columns can significantly reduce memory usage without compromising data integrity.
Another tip is to leverage Pandas’ built-in functions for memory optimization, such as using the `to_numeric` function to convert columns to more memory-efficient data types. Additionally, you can use the `astype` method to explicitly cast columns to the appropriate data types, further reducing memory overhead.
Moreover, consider using the `chunksize` parameter when reading large datasets into Pandas to process data in smaller chunks, which can help avoid memory errors and improve performance. Additionally, utilizing tools like `gc` (garbage collector) to manually release memory can be beneficial in managing memory usage efficiently.
By implementing these memory management tips in Pandas, you can enhance performance, reduce memory overhead, and optimize the processing of large datasets for better productivity and efficiency.
1. Selecting Appropriate Data Types for Columns:
pythonCopy code
import pandas as pd
# Creating a sample DataFrame with large integer columns
data = {
'ID': [1001, 1002, 1003, 1004],
'Value1': [1000000, 2000000, 3000000, 4000000],
'Value2': [10.5, 20.5, 30.5, 40.5]
}
df = pd.DataFrame(data)
# Display the memory usage before optimizing data types
print("Memory Usage Before Optimization:")
print(df.memory_usage(deep=True))
# Optimizing data types for integer columns
df['ID'] = df['ID'].astype('int32')
df['Value1'] = pd.to_numeric(df['Value1'], downcast='integer')
# Display the memory usage after optimizing data types
print("\nMemory Usage After Optimization:")
print(df.memory_usage(deep=True))
2. Leveraging Built-in Functions for Memory Optimization:
pythonCopy code
# Creating a sample DataFrame with string columns
data = {
'Name': ['Alice', 'Bob', 'Charlie', 'David'],
'Category': ['A', 'B', 'A', 'B']
}
df = pd.DataFrame(data)
# Display the memory usage before optimizing data types
print("\nMemory Usage Before Optimization:")
print(df.memory_usage(deep=True))
# Optimizing data types for string columns
df['Name'] = df['Name'].astype('category')
# Display the memory usage after optimizing data types
print("\nMemory Usage After Optimization:")
print(df.memory_usage(deep=True))
3. Using chunksize Parameter for Large Datasets:
pythonCopy code
# Reading a large CSV file in chunks and processing data
chunk_size = 1000
result = pd.DataFrame()
for chunk in pd.read_csv('large_dataset.csv', chunksize=chunk_size):
# Perform operations on each chunk
processed_chunk = chunk.groupby('Category')['Value'].sum()
result = pd.concat([result, processed_chunk])
# Display the processed result
print("\nProcessed Result:")
print(result.head())
4. Utilizing Garbage Collector (gc) for Manual Memory Release:
pythonCopy code
import gc
# Explicitly release memory using gc
gc.collect()
# Display the memory usage after garbage collection
print("\nMemory Usage After Garbage Collection:")
print(df.memory_usage(deep=True))
Explanation:
The first block demonstrates selecting appropriate data types for columns:
- Initially, the DataFrame df has columns 'ID' and 'Value1' with large integer values.
- By using astype() and pd.to_numeric() with appropriate data types ('int32' and 'integer'), the memory usage is reduced.
The second block shows leveraging built-in functions for memory optimization:
- Initially, the DataFrame df has a 'Name' column with string values.
- By converting ‘Name’ to a categorical type using astype('category'), the memory usage is optimized.
The third block illustrates using the chunksize parameter when reading large datasets:
- Reads a large CSV file in chunks of chunk_size rows.
- Performs operations on each chunk (e.g., grouping, aggregation).
- Concatenates the processed chunks to create the final result.
- The fourth block demonstrates using the gc (garbage collector) module for manual memory release:
- Explicitly calls gc.collect() to release memory.
- This can be beneficial in scenarios where large amounts of memory are used and need to be released after processing.
Implementing these memory management tips in Pandas can significantly reduce memory overhead, optimize performance, and improve the efficiency of handling large datasets. Adjust the code according to your dataset size and memory constraints to achieve the best results.
Best Practices for Optimizing Pandas Code for Large Datasets
Optimizing Pandas code for large datasets is crucial for efficient data analysis and processing. When working with massive amounts of data, following best practices can significantly enhance the performance of your code and prevent bottlenecks. Here are some advanced strategies to optimize Pandas code for large datasets:
1. Use Efficient Data Types: Utilize appropriate data types such as int8, int16, float32 instead of int64 and float64 to reduce memory usage and speed up computations.
2. Avoid Iterating Over Rows: Minimize row-wise operations as they are generally slower. Instead, leverage vectorized operations and Pandas built-in functions to process data efficiently.
3. Optimize Memory Usage: Use techniques like downcasting numeric columns, storing categorical data, and removing unnecessary columns to reduce memory usage and improve performance.
4. Utilize Chunking: When working with extremely large datasets that do not fit into memory, consider processing data in smaller chunks using the `chunksize` parameter in Pandas functions.
5. Parallel Processing: Take advantage of parallel processing libraries like Dask or multiprocessing to distribute computations across multiple cores and speed up data processing.
6. Optimize GroupBy Operations: Optimize groupby operations by using `agg` with predefined functions instead of lambda functions and avoid unnecessary sorting.
By implementing these best practices and advanced strategies, you can optimize your Pandas code for large datasets, improve performance, and streamline your data analysis workflows.
Real-world examples and case studies demonstrating the power of Pandas for advanced data manipulation
Real-world examples and case studies are invaluable in showcasing the true power and potential of Pandas for advanced data manipulation. By delving into practical applications, users can gain a deeper understanding of how Pandas can revolutionize their data analysis workflows.
Let’s consider a scenario where a retail company utilizes Pandas to analyze sales data. By leveraging Pandas’ advanced data manipulation capabilities, the company can easily perform tasks such as merging multiple datasets, filtering and sorting data, handling missing values, and aggregating information to derive valuable insights.
For instance, Pandas can be employed to merge sales data with customer demographic information to segment customers based on their purchasing behavior. This segmentation can then be used to tailor marketing campaigns, optimize pricing strategies, and enhance overall customer experience.
Furthermore, Pandas’ ability to handle time series data is instrumental in forecasting sales trends, identifying seasonality patterns, and making data-driven decisions to drive business growth. Through case studies highlighting these real-world applications, users can witness firsthand the transformative impact of Pandas on data manipulation and analysis.
Data Vortex: A Data Science Blog: Series Episode 1 was originally published in Level Up Coding on Medium, where people are continuing the conversation by highlighting and responding to this story.
This content originally appeared on Level Up Coding – Medium and was authored by Chrissie